pwnable.tw部分writeup_Vol.2
( 原文地址:https://0xffff.one/d/469 )
之前在pwnable.tw部分writeup(不定期更新)中已经写了15题了,再继续写就有点乱了,所以另起了一贴。
Alive Note·
是之前Death Note的进阶版,也是写shellcode。
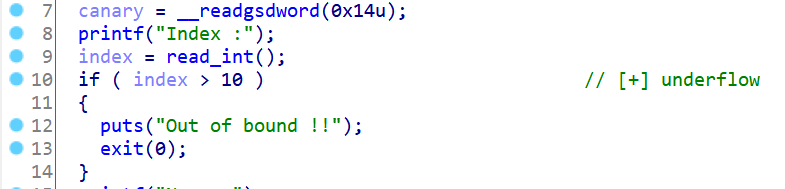
首先在三个功能里都有对输入的index进行上界检查,但没有进行下界检查,所以存在underflow漏洞,覆盖了.got表中某个函数后调用该函数就可以调用shellcode。但问题是shellcode是被分配在堆上的,而且每一块只能8bytes,虽然这个可以用某个jmp绕过,但写起来就有点麻烦了(exp的还算可以,但应该不是最完美的- -)。另外选got表函数时跟Death Note时一样选了free,因为free的参数刚好是某个堆块的地址。做的时候发现可以leak libc函数的方法,虽然在这里因为alnum用处不大。(还有本来以为只用’sh’是可以起shell的,但后来要’/bin/sh’才行,就又更麻烦了)
1 | #!/usr/bin/env python |
BookWriter·
是一个做笔记的程序,漏洞还算是不难找的,
第一个洞是在add里虽然有对现存的笔记数目进行检查,但是范围错了(本来是8页的,但其实可以加9页),所以最后溢出了一页会覆盖掉第0页的大小值为一个堆上的地址,是一个非常大的值,然后再edit里就可以对第0页进行没有大小限制的修改,覆盖其他的堆块。
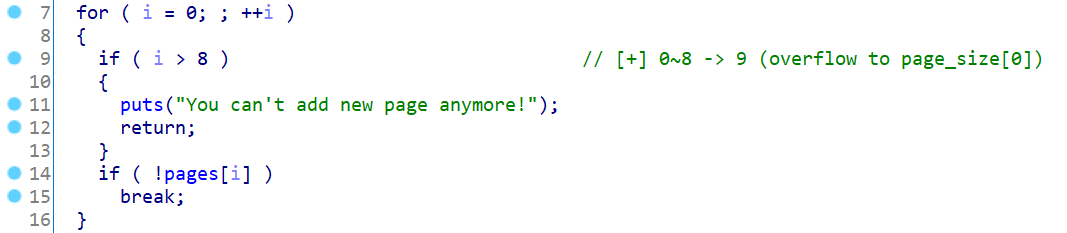
第二个是输作者名字时如果刚好输够64bytes(反正就是末尾不是\x00或\n)的话就没有进行零截断,可以利用来泄露堆地址。

找到两个洞后还以为这题怎么这么容易,后来发现堆题竟然没有free?后来找了一下,发现有一种叫House of orange的做法(其实只用了方法中的一半,后面因为用到了FSOP有点复杂,而这题又更简单的解法,所以就没用FSOP了)。
首先是在malloc的时候,如果发现top chunk太小的话,会用mmap(应该是mmap)的方法分配一块新的空间用作top chunk,然后旧的top chunk就会被放入unsorted bin中,上面也说到堆中的东西可以在第0页被edit时覆盖掉,所以top chunk的大小和分配新top chunk后的old top chunk的bk是可以被修改的,所以就可以在author name里造一块假的chunk后利用unsorted bin attack覆盖author name以及它后面的page的地址和page size(注意虽然add是可以加9页的,加满后重新edit第0页输入空后第0页的size就变0了,就可以再加一页),覆盖page地址后就可以利用edit和view进行任意位置读写了。
先提一下top chunk重新分配那个机制,top chunk的分配是调用sysmalloc函数的(源码再malloc.c),sysmalloc会对old chunk(就是被我改小的那个)的大小进行检验,具体在一个assert中(看图)。第一个好像是大小为0的情况,即应该是改的大小不能为0;第二串有几个检查,首先是old_size(即改的那个)不能小于MINSIZE,MINSIZE测过大概是0x1f左右(也不知道又没看错,反正别太小就好了)。第二个是prev_inuse位即最低位要是1。第三个是old_end要页对齐,即old top chunk的地址加上size的低24位是0(4K对齐)。如果上面其中一个不满足的话会报错。
最后可以任意读写了就随便搞都可以了,通过读libc里envion的内容可以得到stack的地址,然后叠个ROP,搞定。
1 | #!/usr/bin/env python |
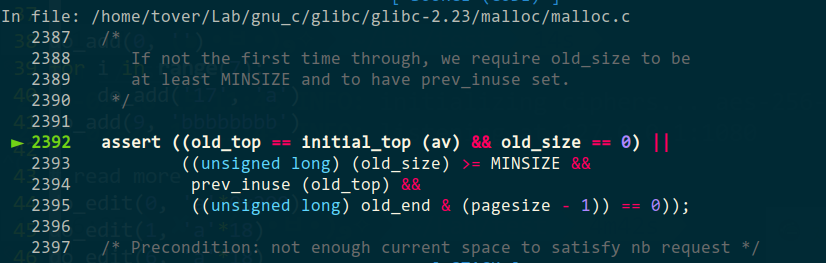
CAOV·
题目很好心的给了源码,看了两遍找不到洞在哪,然后直接上fuzz,大概十几分钟就有结果了,然后才发现源码是用来坑人的。。。
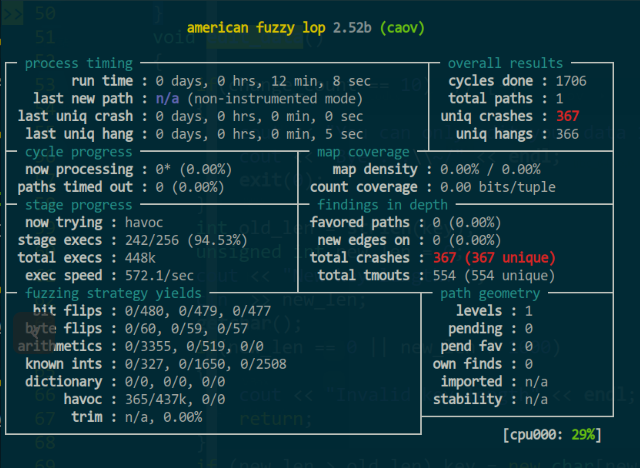
fuzz的结果大概是在edit的时候如果名字输很长的话就会crash,原因在于C在执行赋值运算符重载时除了传入左值和右值外还会传入一个不可名状的局部变量(少接触C题,暂时还不知道是什么机制,查到了再补,咕),就是下图的v2(用IDA打开很容易可以看到,所以源码是拿来坑人的- -),v2被传入赋值重载函数(图中D_operator_copy)后是直接返回的,所以这个可以不用管;问题在于v2用完后会被析构(图中的D_destructor),但在这之前v2是没被构造的,而且v2是没有初始化的局部变量,它的值会受到上一个函数(就是set_name)在栈中保留的值影响。所以最后控制好v2的值的话就可以实现任意地方的free。
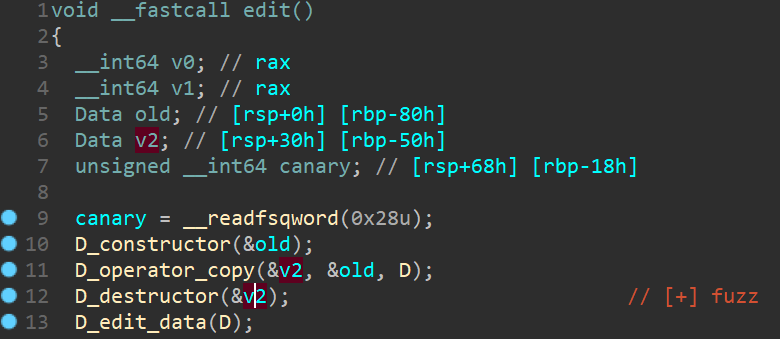
先明确一下可以实现malloc的只有分配新的key时,可以实现free的只有Data的析构。因为Data D是放在堆上的,所以利用思路是控制堆上D的key,实现往key中写入任意地址的话就可以实现任意地方的读和大部分地方的写(写的长度不能大于原来空间字串长度)。
首先要拿到heap的基址,因为可以任意free所以就是用fastbin attack了,需要先free一个堆上的chunk,然后在.bss的name上构造假chunk再free掉,再分配对应大小的块就可以把key分配到name上,且刚好是fd的值(思路就是这样了,实现起来还是很有点痛苦- -)。至于第一步的堆块怎么free的话,因为程序在edit时会保存一个old的Data,edit完后old会被析构,就可以free一个edit前的key大小的chunk(刚开始的时候key是在heap上的)。
然后是控制Data D,经操作后发现第一次输入的name是在堆上的,而且位置非常好,刚好在Data D上,所以就可以利用这个name在堆上先构造个fake chunk header,然后利用上面同样的fastbin attack方法就可以把chunk分配到这里,输入的key值就可以覆盖Data D。

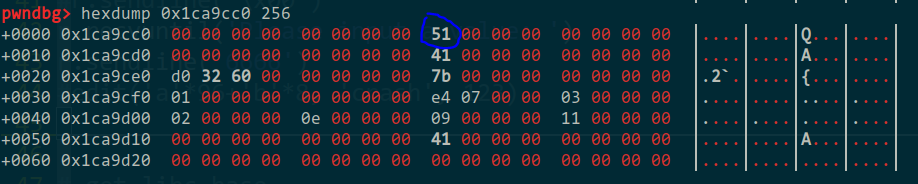
到这里就实现了任意读和基本任意写了,然后就可以随便搞了,本来是可以改malloc_hook之类的,但hook的初始值都是0写不了,而且写栈还是最稳的,所以就写栈了。首先读.got可以拿到libc_base,读libc中的environ可以拿stack地址,然后写main的返回地址就好(因为main的返回地址可以写6bytes)。经实验,发现main返回地址后的内容是0,所以只能写一个地址(在本地跑是可以多个的。。。),然后就one gadget,getshell。
1 | #!/usr/bin/env python |
另外因为开始写的时候是用本地一个自己编的libc做测试的,在那个libc中调用update_time后会分配一个很大的chunk,然后调用malloc_consolidate把fastbins合并到unsorted bin中,还有一大堆条件要绕(非常痛苦),最后写完后换题目库时发现并没有这个操作,所以就要又重写了一份- -(非常绝望),既然写都写了就把exp也放一下吧,主要通过fastbin attack覆盖.bss上的stdout位fake_fp。
1 | #!/usr/bin/env python |
这大概是至今为止做过的exp写最长、writeup写最长、flag也最长的题目了- -